I love talking with Ryan and others passionate about new technology and languages. Lately though I have been increasingly jaded with anything ‘new’.
How many different data technologies do we need? ADO .Net, Nhibernate, Linq-2-SQL, Entity Framework version.. whatever. We have been connecting to databases for over 20 years so why are we still worrying about this?!
The sheer number of UI frameworks is reaching the point of ridiculousness. I was reading the WPF Disciples Google Group and ran across this post. Paul Stovell listed all the MVVM, MVC, MVC frameworks he knew of (around 11). Then everyone was commenting with their own ‘me too’ response.
Am I the only one who finds that utterly hilarious? How many frameworks do we need?!
Ryan is extremely involved with Ruby, Python, F#, and the other ‘new’ languages to the .Net Framework. I have been delaying learning Ruby for too long, because it’s hard to get motivated to learn a new language. I see the potential and the possibilities, but I quickly get discouraged and uninterested.
I have to ask myself… why am I jaded? Why am I disinterested in things that used to excite me?
The more I thought about it, the more the conclusion became crystal clear. Every project I have been a part of has had the same problems. None of the problems were technology related.
Why would I get excited about a solution to a problem I don’t have?
Reminds me of Karl Pilkington’s comment about this picture of twins on one of the Ricky Gervais podcasts. Karl saw a picture of a set of twins on an office desk (obviously the kids of the guy sitting at the desk). His great idea was since they look alike and are dressed alike, the parent could just have the picture of one of them and save space. Ricky Gervais then commented something like :
What you have there is the best non-solution to a problem that doesn’t exist.
And that is pretty much how I feel about most of the technological innovations these days.
For example: can someone explain to me how Oslo is going to help me? I am also not sold on RIA or anything Cloud related. The technology is interesting, but I can’t justify where I would use any of this stuff.
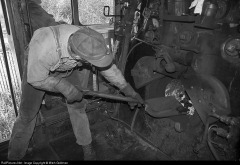
Sometimes I feel like a lone engineer shoveling coal into a furnace on a runaway train. I don’t know where we are going, where we have been, and all I hear is FASTER, FASTER!
When I scream for help, I get delivered a better shovel.
I don’t want a better shovel, I want to know why I am shoveling so much coal!!!
Can’t we do more with less? Can’t we slow down? Do we even know why we are in this train?!
Lately the murmurings on different blogs, podcasts, and developers has been that software development has gotten too complicated. How can that be true when we have tons of tools and frameworks that should make life easier? jQuery makes Javascript easier. Pick your favorite Data Access tool / ORM makes data access easier. Any number of UI frameworks makes UI construction easier. So why do we think things are more complicated? Shouldn’t our life be easier?
The majority of projects are always behind, always over budgeted, and always unclear on where they are and where they are going. This has been my experience spanning multiple companies and multiple positions on different teams. I am fully humble enough to recognize that “I” might be the problem, but I doubt it. There is enough shared pain through my conversations with other developers to recognize its a industry wide problem.
The only project I was a part of that didn’t feel this way had a full time Project Manager and a team full of very skilled developers. To me it didn’t feel like the project was better run, it was just we had enough skilled team members to overcome any poor execution or poor planning. That isn’t saying our PM wasn’t an all star because he was. My point is that although the project was a ‘success’ I didn’t feel like it was successful because we had a great methodology. We just powered through any complications.
Every project (including that last ‘successful’ one) always had mad dashes of insane hours of work followed by listless lulls as we waited for one thing or another. I never felt I had enough time to do my work and other activities related to work (ie blog, present, create frameworks)
Where am I going with this? I wanted to capture my state of mind and why I am re-examining how and why I develop.
The status quo will burn me out.
The ole definition of insanity : Doing the same thing over and over again expecting different results.
I am tired of doing the same thing, so what can I do different? Why is there this constant pain? I know there are solutions and it’s time for me to document my mental journey as I search for answers. Maybe my breadcrumbs will help those that follow.